Nest.js | Dockerfile
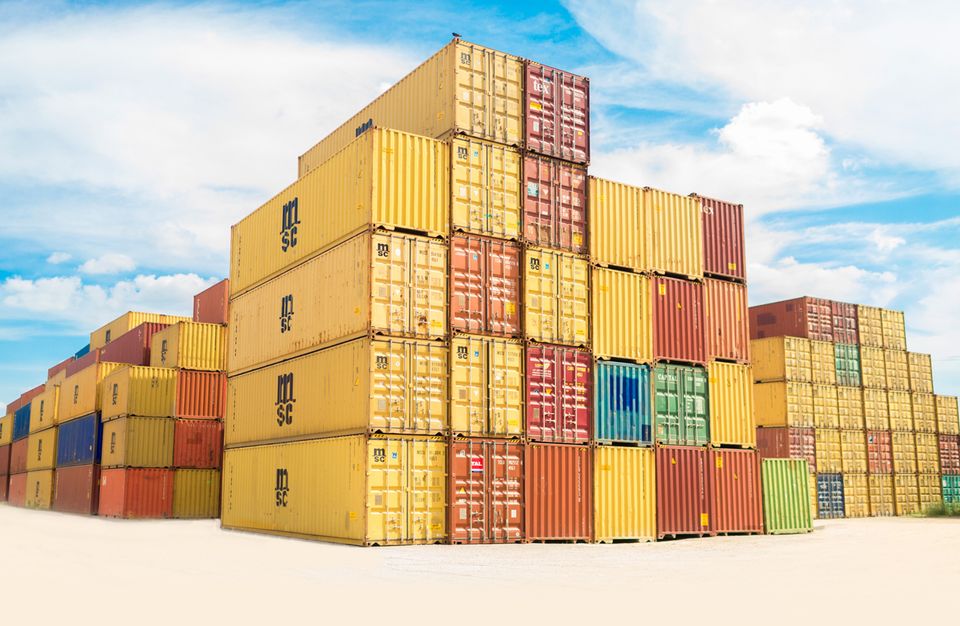
Below is an example of a Dockerfile for a Nest.js framework application. This assumes you have a basic understanding of Docker and its concepts.
!!!WE URGE TO STOP DOING THIS!!!
# Use a base image with Node.js installed
FROM node:latest
# Create and set the working directory inside the container
WORKDIR /usr/src/app
# Copy package.json and package-lock.json to the container
COPY package*.json ./
# Install application dependencies from package-lock
RUN npm install
# Copy the application source code to the container
COPY . .
# Expose the port that the application runs on (change as needed)
EXPOSE 3000
# Command to start the Nest.js application
CMD ["npm", "run", "start:dev"]
FROM node:latest
: This line specifies the base image for the container, using the latest Node.js image available from the official Docker Hub repository.WORKDIR /usr/src/app
: Sets the working directory within the container where the application code will be placed.COPY package*.json ./
: Copies the package.json and package-lock.json files to the container. This allows Docker to take advantage of layer caching for faster builds by only rebuilding the necessary layers when dependencies change.RUN npm install
: Installs the application dependencies defined in the package.json file within the container.COPY . .
: Copies the entire application source code to the container. This assumes that your Nest.js application code is in the same directory as the Dockerfile.EXPOSE 3000
: Informs Docker that the application running inside the container will be accessible on port 3000. Modify this according to your Nest.js application's specified port.CMD ["npm", "run", "start:dev"]
: Defines the command to start the Nest.js application. Modify this command as necessary based on how you start your Nest.js application (e.g.,npm run start
,npm run start:dev
, etc.).
To build your Docker image, navigate to the directory containing this Dockerfile and run:
docker build -t your-app-name .
Replace your-app-name
with the name you want to give to your Docker image. After the build, you can run a container based on this image using docker run
with appropriate port mapping and additional configurations as needed.
Result:
IMAGE ID SIZE
452c9f7218ae 598MB
Multi-stage builds are useful to anyone who has struggled to optimize Dockerfiles while keeping them easy to read and maintain.
!!!DO THIS!!!
# ---- Base Node ----
FROM node:20.8.1-bullseye-slim AS base
WORKDIR /usr/src/app
# Install app dependencies
COPY package*.json ./
RUN npm install
COPY . .
# ---- Build ----
FROM base AS build
RUN npm run build
# ---- Production ----
FROM node:20.8.1-bullseye-slim AS production
WORKDIR /usr/src/app
COPY --from=build /usr/src/app/dist ./dist
COPY package*.json ./
RUN npm ci --only=production
CMD ["node", "dist/main"]
- Base Node (base):
- Sets up a base stage with Node.js and defines a working directory.
- Copies the package.json and package-lock.json files to install app dependencies.
- Copies the application source code into the container.
- Build (build):
- Inherits from the base stage.
- Executes the build process for the application. For a Nest.js app, this might involve compiling TypeScript code into JavaScript (or any other build tasks required).
- Production (production):
- Uses a smaller image (node:20.8.1-bullseye-slim) to reduce the final image size.
- Sets the working directory and copies only the necessary files for production (the built output from the previous stage).
- Installs only production dependencies using
npm install --only=production
. - Specifies the command to start the application in production mode (
node dist/main
assuming the main entry point file ismain.js
in thedist
directory after building).
To build your Docker image, navigate to the directory containing this Dockerfile and run:
docker build -t your-app-name .
Result:
IMAGE ID SIZE
732d8ffb3f3b 261MB
That's not finally solution, but it's a good start point for your project.
Please check our story about choosing Docker image for Node.js:

Member discussion